Randomise an Array
This is a very common activity. You might need to adjust the order of some choices or other data you are manipulating.
var aData = [1, 2, 3, 4, 5, 6];
wscScripting.randomizeArray(aData);
// E.g [3, 2, 5, 4, 6, 1]
This will randomly sort the the data in the array. The data held in aData will be randomised
Sort an Array of Numerics
You might need to adjust the order of some numbers to ensure the numbers selected are in ascending or descending order
var aData = [10, 2, 7, 5, 1, 4];
wscScripting.sortNumericArray(aData, true);
// E.g [1, 2, 4, 5, 7, 10]
This will sort the the data in the array. The data held in aData will be sorted. Changing the 2nd parameter to false will sort the array in descending order.
Sort an Array of Strings
You might need to adjust the order of some pieces of text to ensure the text selected are in ascending or descending order
var aData = ['Ten', 'Two', 'Seven', 'Five', 'One', 'Four'];
wscScripting.sortStringArray(aData, true);
// E.g ['Five', 'Four', 'One', 'Seven', 'Ten', 'Two']
This will sort the the data in the array. The data held in aData will be sorted. Changing the 2nd parameter to false will sort the array in descending order.
Get First Ranked Choice in a Ranking Question
You might need to find which choice was selected first
// Assumes there is a Question with a Data Access Code of 'SOURCE'
var oQuestion = wscScripting.getQuestionByDataPipingCode('SOURCE');
var aRanks = wscScripting.getSelectedRanks(oQuestion);
if (aRanks && aRanks.length > 0) {
var oChoice = aRanks[0];
// e.g. Choice Text is oChoice.text
}
This will obtain the question and then query the choices that may have been ranked. Once done, you can then look at the top selected choice.
Select choices in a Question based on selections in a previous Question based on their value
This is a very common activity. You have a question where you have some choices selected and wish to select similar choices in a subsequent question. You might do this to aggregate selections from multiple questions.
// Assumes there are 2 Questions with a Data Access Codes of 'SOURCE' and 'TARGET'
// Both questions have choices with the same values
var oSource = wscScripting.getQuestionByDataPipingCode('SOURCE');
var oTarget = wscScripting.getQuestionByDataPipingCode('TARGET');
if (oSource && oTarget) {
var aSelected = wscScripting.getSelectedChoices(oSource);
if (aSelected.length > 0) {
for (var nChoice = 0;nChoice < aSelected.length;nChoice++) {
wscScripting.selectChoiceByValue(oTarget, aSelected[nChoice].value);
}
}
}
This will obtain the selected choices for the SOURCE question and then select the choice in the TARGET question based on the same value.
Select choices in a Question based on selections in a previous Question based on a TAG called CODE
Sometimes you might have a question where you have some choices selected and wish to select similar choices in a subsequent question. If both questions do not have the same choice values you'll need to use TAGS to find the right choice to select.
// Assumes there are 2 Questions with a Data Access Codes of 'SOURCE' and 'TARGET'
// Both questions have choices which use TAGS with a TAG name of CODE
var oSource = wscScripting.getQuestionByDataPipingCode('SOURCE');
var oTarget = wscScripting.getQuestionByDataPipingCode('TARGET');
if (oSource && oTarget) {
var aSelected = wscScripting.getSelectedChoices(oSource);
if (aSelected.length > 0) {
for (var nChoice = 0;nChoice < aSelected.length;nChoice++) {
var oChoice = aSelected[nChoice];
var oTag = wscScripting.getTagValueByName(oChoice.tags,'CODE');
if (oTag) {
var oTargetChoice = wscScripting.getChoiceByTagValue(oTarget, 'CODE', oTag.text);
if (oTargetChoice) {
wscScripting.selectChoice(oTarget, oTargetChoice);
}
}
}
}
}
This will obtain the selected choices for the SOURCE question and then select the choice in the TARGET question based on the choices in both questions having the same TAG values for the TAG called CODE
Create a custom validation and validation message
Sometimes you might have a need to validate a question based on very specific criteria. This could include specific business logic. For example, checking postal codes.
This script shows validating a Text Question for specific values. The principal can then be applied to any question.
This script should be placed in the Execute Javascript before Survey Page is Validated scripting event.
// Assumes a Text Question with a Data Access Code of 'SOURCE'
var oQuestion = wscScripting.getQuestionByDataPipingCode('SOURCE');
if (oQuestion) {
var sValue = wscScripting.getValue(oQuestion);
// Test the value here
// For example ensure the value entered is 'ONE' or 'TWO'
if (sValue == 'ONE' || sValue == 'TWO') {
// This value is valid
wscScripting.clearValidation(oQuestion);
args.isValid = true;
}
else {
// This value is not valid
wscScripting.setValidation(oQuestion, 'You must enter a valid value');
args.isValid = false;
}
}
This will check the value from the question and compare it to valid values. If it succeeds, it clears any validations that might exist. If it doesn't succeed, it sets a validation message for the question.
The message will appear similar to other validation messages for questions.
Get a Url Parameter. That is, a Query String
Sometimes you might have a link to a survey and have additional parameters passed. For example, this can happen when you use Panel Providers. To get access to these parameters in script you can utilise the getUrlParameter function.
var sParameter = wscScripting.getUrlParameter('lang');
// E.g sParameter == 'en'
This will obtain a language code passed into the survey.
Load Custom CSS
Sometimes you might have a need to use custom style sheets (CSS) to maintain a client's corporate style for their survey.
You can use Themes to load custom external CSS or you could might want to load it via JavaScript
$('head').append(
$('<link rel="stylesheet" type="text/css" />')
.attr('href','https://fonts.googleapis.com/css?family=Lobster&subset=latin,latin-ext') );
This will load a font from the Google Font API and make it available in your markup.
Select Choices based on "Least Filled" Quotas
Sometimes you need to have your survey react in very specific ways to Quotas so that different pathways can be taken based on how many quotas have been filled.
You can use scripting to review the Quotas and then select choices or undertake other actions. Selecting choices might allow additional Quotas to be calculated or Flow Control to operate based on current Quota calculations.
This script finds the "Least Filled" Quotas and then based on the Quota Codes selects choices in a Choice question.
var nNumberToSelect = 3;
var oQuestion = wscScripting.getQuestionByDataPipingCode('QUOTACHOICES');
// Get the quotas
var aQuotas = new Array();
aQuotas.push(wscScripting.getQuotaByCode('QUOTA1'));
aQuotas.push(wscScripting.getQuotaByCode('QUOTA2'));
aQuotas.push(wscScripting.getQuotaByCode('QUOTA3'));
aQuotas.push(wscScripting.getQuotaByCode('QUOTA4'));
aQuotas.push(wscScripting.getQuotaByCode('QUOTA5'));
// Shuffle the quotas first
wscScripting.randomizeArray(aQuotas);
// Sort the list to be in least responded order
wscScripting.sortQuotasByLeastFilled(aQuotas);
// Select choices while we have least filled to a maximum of nNumberToSelect
for(var nQuota = 0;nQuota < aQuotas.length;nQuota++) {
var oQuota = aQuotas[nQuota];
if (wscScripting.getSelectedChoices(oQuestion).length >= nNumberToSelect) {
// Do not select any further
break;
}
// Find a choice that has a code that matches the quota code and select it
var oChoice = wscScripting.getChoiceByTagValue(oQuestion, 'CODE', oQuota.code);
if (oChoice) {
wscScripting.selectChoice(oQuestion, oChoice);
}
}
This will pick the least filled quotas up till a maximum limit and then select the same choices based on their TAG.
Connect to a VoxPopMe project
For those users who want to connect their survey to a VoxPopMe project you will need to follow the VoxPopMe guide for building the embedded script.
The following script is an example of how this could be achieved. The code is available as-is with no guarantee of it's ability to work with current versions of VoxPopMe.
$('#{QuestionContainer}').addClass('wsc_question-zone');
$('#{QuestionContainer}').html('<div class="wsc_question-body" id="xx"><div class="vpm-capture-widget"></div></div>');
vpm_widget_config = {
project_id: "put-you-voxpopme-project-id-here",
additional_data: {
'first_name': 'Xxxxx',
'userid': wscScripting.getRecallCode()
},
settings: {
locale: "en-GB"
},
disable_next_button: function() {
// Insert disable 'Next' button function appropriate to your survey platform here
$('#nextbutton').hide();
$('#submitbutton').hide();
},
enable_next_button: function() {
// Insert enable 'Next' button function appropriate to your survey platform here
$('#nextbutton').show();
$('#submitbutton').show();
}
};
!function(a,b,c,d){function e(){var a=b.createElement(c);a.type="text/javascript", a.async=!0,a.src=d;var e=b.getElementsByTagName(c)[0]; e.parentNode.insertBefore(a,e)}a.attachEvent?a.attachEvent("onload",e):a.addEventListener("load",e,!1)}(window,document,"script","https://capture.voxpopme.net/main.js");
document.addEventListener("DOMContentLoaded", function(event) {
// Replace your_NextButton below with the appropriate function
// to disable 'Next' button in your survey platform
document.getElementById("nextbutton").style.display = "none";
// Replace your_checkbox is your survey platform's class for selection a checkbox
$('.wsc_checkfield input').change(function() {
// click checkbox?
if ($(this).is(':checked')) {
// Insert enable 'Next' button function appropriate
// to your survey platform here
$('#nextbutton').show();
} else {
// Insert disable 'Next' button function appropriate
// to your survey platform here
$('#nextbutton').hide();
}
});
});
args.isValid = true;
Using a Range Slider to hide and show questions
Sometimes you need to allow a slider to show additional questions dependant on their values. For example, you might have a range from 1 to 100, but if the value exceeds 50 you want to show some additional questions.
You can use a small script to add an event for the slider that will react to changes in the slider value and allow you to hide or show other question.
var oQuestion = wscScripting.getQuestionByDataPipingCode('CONTENTTOSHOW');
// Create the event once the slider has rendered
$(document).ready(function() {
var oSlider = wscScripting.getQuestionByDataPipingCode('SLIDER');
var oRange = $('#' + oSlider.identity + '_range').data("ionRangeSlider"));
oRange.update({
onChange: function (data) {
// fired on every range slider update
var oContent = wscScripting.getQuestionByDataPipingCode('CONTENTTOSHOW');
console.log(data.from, data.to);
if (data.from > 50 && data.to < 60) {
wscScripting.showQuestion(oContent);
}
else {
wscScripting.hideQuestion(oContent);
}
}
});
});
Time how long it takes for a user to select a choice on single and multiple choice questions
Sometimes you may need to time how long it takes for a user to select a choice in a single or multiple choice question.
This script will time how long it takes for the user to click/select a choice after the page has been loaded, it requires two hidden Numeric questions.
Two time values will be set into the numeric fields, the first will be the start time after the page has loaded. The second will be the amount of time it took for a user to click on a choice. Currently this will not work on Matrix style questions.
$(document).ready(function () {
//Get start time after the page has loaded;
var nStartTime = performance.now();
//Get the start time question
var oQuestionStart = wscScripting.getQuestionByDataPipingCode('Q1_START');
//Strip milliseconds and convert to 4 decimal places
nStartTime = nStartTime /= 1000;
nStartTime = nStartTime.toFixed(4);
//Set the start question with start time
wscScripting.setValue(oQuestionStart, nStartTime);
//Grab the choice question
var oQuestion = wscScripting.getQuestionByDataPipingCode('BRAND');
//Loop through the choices and check if a choice was clicked
for (var nChoice = 0; nChoice < oQuestion.choices.length; nChoice++) {
var oChoice = oQuestion.choices[nChoice];
$('#' + oQuestion.identity + '_' + oChoice.identity + '_table').on('click', function () {
var endTime = performance.now();
endTime = endTime /= 1000;
endTime = endTime.toFixed(4);
//Get the start time question
var oQuestionStart = wscScripting.getQuestionByDataPipingCode('Q1_START');
//Get the end time question
var oQuestionTime = wscScripting.getQuestionByDataPipingCode('Q1_TIME');
//Check if the value of the end time question field is already filled
if (isNaN(wscScripting.getValue(oQuestionTime))) {
//Get the start time value from start time question
var nStartTime = wscScripting.getValue(oQuestionStart);
//Calculate the end time - start time
var nFinalTime = endTime - nStartTime;
nFinalTime = nFinalTime.toFixed(4);
//Update the end time text field with how long it took the user to click a choice
wscScripting.setValue(oQuestionTime, nFinalTime);
}
});
}
});
This script should be placed in the Execute Javascript before Survey Page is Validated scripting event.
Select a question choice based off a specific age value from a numeric question
You may need to select a specific choice in question based on what a user inputs as their age in a numeric question.
This script will select one of two choices in a hidden question depending on what age was input into a numeric question.
//Get the questions
var oQuestion = wscScripting.getQuestionByDataPipingCode('AGE');
var oHiddenQuestion = wscScripting.getQuestionByDataPipingCode('HQ');
//Get the age selected
var nAge = wscScripting.getValue(oQuestion);
//Set the condition for the persons age
if (nAge >= 15 && nAge <= 17){
//Select code 1
wscScripting.selectChoiceByValue(oHiddenQuestion, 1);
} else if (nAge >= 18 && nAge <= 19){
//Select code 2
wscScripting.selectChoiceByValue(oHiddenQuestion, 2);
};
Hide or show a question based on a checkbox that has been clicked
You may want to hide or show a particular question on your survey page.
This script requires a checkbox question with one choice. When the checkbox is clicked, a chosen question on your
survey page will be hidden. When the checkbox is clicked again, the question will be shown again.
//Get question 1
var oQuestion1 = wscScripting.getQuestionByDataPipingCode('Q1');
//Add an onclick event to the checkbox
$('#' + oQuestion1.identity + '_' + oQuestion1.choices[0].identity + '_table').on('click', function (){
//Get question 1 and 2
var oQ1 = wscScripting.getQuestionByDataPipingCode('Q1');
var oQ2 = wscScripting.getQuestionByDataPipingCode('Q2');
//Check if the checkbox has been clicked
if (wscScripting.isChoiceSelectedByValue(oQ1, 1) == true) {
//if the checkbox is checked, hide question 2
wscScripting.hideQuestion(oQ2);
} else {
//if the checkbox is not checked, show question 2
wscScripting.showQuestion(oQ2);
}
});
Random Selection Of Choices Across Multiple Choice Questions - Choices Can Only Be Picked A Limited Amount Of Times
This script can be used to randomly select a number of choices across multiple questions, however
each choice selected can only be chosen a certain number of times. There are three primary functions for this script: reset(), doRandomization() and checkRandomization().
The amount of times a choice can be chosen can be modified.
For example:
aChoicesRemaining.push(3); and aChoicesRemaining[i] = 3;
can both be changed to the integer 4 to allow each choice to be picked a maximum of 4 times instead of 3.
The code
if (wscScripting.getSelectedChoices(aQuestions[i]).length >= 4)
in the doRandomization function can also be adjusted to how many choices per question you want to be selected. This could be increased for example to 5 if you wanted 5 choices per question selected.
You can click the following link for a demonstration of the script in action:
Randomisation Example
Note in the demonstration above there is four multiple choice questions with ten choices per question. Choices will be selected at random, but each choice will not be selected more than three times, you can refresh the page to observe the different choices selected each time.
When using this script it is important to note that the amount of questions and choices per question you want to use can impact the randomisation.
//Setup function - when called - will reset all the questions and the ChoicesRemaining array
function reset(aQuestions) {
//Loop through each question and reset it
for (i = 0; i < aQ.length; i++) {
wscScripting.resetQuestion(aQ[i]);
};
//Reset the choices tally in the aChoicesRemaining array
for (i = 0; i < aChoicesRemaining.length; i++) {
//The number of allowed choices is set back to 3 for each choice
aChoicesRemaining[i] = 3;
};
};
//Setup function - when called will loop through each question, randomize the choices for the question
//The function is currently setup to check if 4 or more choices have been selected
//If a choice is selected, -1 for that choice will be taken away in the ChoicesRemaining array
function doRandomization(aQuestions) {
//Randomize array of questions
wscScripting.randomizeArray(aQuestions);
//Loop through each question
for (i = 0; i < aQuestions.length; i++) {
//Randomize the choices of the question
wscScripting.randomizeArray(aQuestions[i].choices);
//Loop through the choices
for (j = 0; j < aQuestions[i].choices.length; j++) {
//Check if the question already has 4 or more choices selected
if (wscScripting.getSelectedChoices(aQuestions[i]).length >= 4) {
//Break out of the loop if 4 or more choices are already selected - process will be repeated for the
//next question
break;
};
//Get the ordinal position of the choice in the aChoicesRemaining array by using the choice value
//If the tally for the choice in aChoicesRemaining hasn't reached 0 yet, we can still use that
//choice in our question
if (aChoicesRemaining[aQuestions[i].choices[j].value - 1] > 0) {
//When a choice is selected, -1 for that choice in the aChoicesRemaining array, we don't want to
//pick the same choice more than 3 times as the aChoicesRemaining array has been set to a maximum of 3 selections per choice
aChoicesRemaining[aQuestions[i].choices[j].value - 1]--;
wscScripting.selectChoice(aQuestions[i], aQuestions[i].choices[j]);
};
};
};
};
//Setup function - used to check all the questions after randomization has been done
//Checks and compares question choices for duplicate patterns
function checkRandomization(aQuestions) {
//Check each choice is selected
aChoicesRemaining.forEach(function (e) {
if (e > 0) {
return false;
};
});
//Check for duplicate choice patterns
for (i = 1; i < aQuestions.length; i++) {
//Select first question choices
var selectedChoicesA = wscScripting.getSelectedChoices(aQuestions[i]);
for (j = i - 1; j >= 0; j--) {
var bMatched = true;
//Select second question choices
var selectedChoicesB = wscScripting.getSelectedChoices(aQuestions[j]);
//Begin testing choices for duplicates
if (selectedChoicesA.length == selectedChoicesB.length) {
for (x = 0; x < selectedChoicesA.length; x++) {
var bFound = false;
for (z = 0; z < selectedChoicesB.length; z++) {
if (selectedChoicesB[z].value == selectedChoicesA[x].value) {
bFound = true;
break;
};
};
if (!bFound) {
bMatched = false;
break;
};
};
};
if (bMatched) {
return false;
};
};
};
//Return true if no duplicates are found
return true;
};
//Grab your questions and push them into the aQ array
var aQ = new Array();
aQ.push(wscScripting.getQuestionByDataPipingCode('SOURCE_1'));
aQ.push(wscScripting.getQuestionByDataPipingCode('SOURCE_2'));
aQ.push(wscScripting.getQuestionByDataPipingCode('SOURCE_3'));
aQ.push(wscScripting.getQuestionByDataPipingCode('SOURCE_4'));
//The aChoicesRemaining array is used to keep track of how many times a choice is selected across your questions
//Currently, we don't want a choice to be selected more than 3 times
var aChoicesRemaining = new Array();
aQ[0].choices.forEach(function (e) {
//The number of times a choice can be selected across your questions can be changed by adjusting the
//push() integer
aChoicesRemaining.push(3);
});
//Reset all the questions in the aQ array
reset(aQ);
//Randomize the choices and select choices for each question
doRandomization(aQ);
//Continue looping through all the selected choices and continue checking until there is no duplicate patterns
while (!checkRandomization(aQ)) {
//If checkRand returns false, a duplicate pattern is found, reset all the questions
reset(aQ);
//Because a duplicate pattern was found, randomize again
doRandomization(aQ);
};
This script should be placed in the Execute Javascript when Survey Page has Loaded scripting event.
Pipe Video Collection Setup Script
The following script can be used as a template to setup video recording in your survey and have it uploaded to
addpipe.com
You will need to fill in the parameters
jobId, eid, and
accountHash with the required information for the script to function correctly.
Other parameters such as the width, height, and video quality can also be adjusted to your needs. You can see in this example the video quality is currently set to 720p.
var oLink = document.createElement('link');
oLink.type = "text/css";
oLink.rel = "stylesheet";
oLink.href = "https://cdn.addpipe.com/2.0/pipe.css";
oLink.onload = function () {
var oScript = document.createElement('script');
oScript.type = 'text/javascript';
oScript.src = 'https://cdn.addpipe.com/2.0/pipe.js';
oScript.onload = function () {
var pipeParams = {
size: {
width: 640,
height: 390
},
qualityurl: "avq/720p.xml",
accountHash: "put-your-account-hash-here",
eid: "put-your-eid-here",
mrt: 120,
payload: "userId: [@RECALLCODE@], jobId: put-your-job-id-here"
};
PipeSDK.insert("{QuestionContainer}",pipeParams,function(recorderObject){});
};
document.getElementsByTagName('head')[0].appendChild(oScript);
};
document.getElementsByTagName('head')[0].appendChild(oLink);
You must have Show a Content Container for this Javascript to use enabled for this script in WSC. This script should be placed in the Execute Javascript when Survey Page has Loaded scripting event.
Pipe Video with Validation
You may need video recording/uploading to be mandatory for respondents, this can be tricky as the addpipe video player is rendered through Javascript rather than a question in WSC.
This can however be achieved by hooking into specific addpipe video events using the addpipe Javascript Events API.
The following scripts can be used to force respondents to record/upload a video on desktop and mobile devices before being able to proceed in the survey.
For the scripts to function correctly, you will need a hidden choice question on the page which will be used to validate another visible question, EG a text question.
Event listeners will be added to the recorder object and will automatically select a choice in the hidden question when an event is triggered. A validation script will then be used to check the hidden question during the Survey Page is Validated scripting event.
You can read more about the Pipe Video Recorder Event API here:
Pipe Video Event API
//Validation Script
//Get the questions
var oQText = wscScripting.getQuestionByDataPipingCode('TEXTQUESTION');
var oQCheckUpload = wscScripting.getQuestionByDataPipingCode('CHECKUPLOAD');
//Check if YES is selected in the hidden question
if (wscScripting.isChoiceSelected(oQCheckUpload, oQCheckUpload.choices[0]) == true) {
//If yes is selected, pass the validation on the text question
wscScripting.clearValidation(oQText);
args.isValid = true;
} else {
//No is selected, fail the validation
wscScripting.setValidation(oQText, 'You MUST upload or record a video');
args.isValid = false;
};
//Pipe Video Script
//Get the questions
var oQText = wscScripting.getQuestionByDataPipingCode('TEXTQUESTION');
var oQCheckUpload = wscScripting.getQuestionByDataPipingCode('CHECKUPLOAD');
//Set the hidden question to false upon entering the survey page
wscScripting.selectChoice(oQCheckUpload, oQCheckUpload.choices[1]);
var oLink = document.createElement('link');
oLink.type = "text/css";
oLink.rel = "stylesheet";
oLink.href = "https://cdn.addpipe.com/2.0/pipe.css";
oLink.onload = function () {
var oScript = document.createElement('script');
oScript.type = 'text/javascript';
oScript.src = 'https://cdn.addpipe.com/2.0/pipe.js';
oScript.onload = function () {
var pipeParams = {
size: { width: 640, height: 390 },
qualityurl: "avq/720p.xml",
accountHash: "put-your-account-hash-here",
eid: "put-your-eid-here",
mrt: 120,
sis: 0,
asv: 1,
mv: 0,
dpv: 0,
ao: 0,
dup: 1,
payload: "userId: [@RECALLCODE@], jobId: put-your-job-id-here",
};
PipeSDK.insert("{QuestionContainer}", pipeParams, function (recorderInserted) {
//Add event listeners
//DESKTOP event fires when video RECORDING UPLOAD is complete
recorderInserted.onUploadDone = function(recorderId, streamName, streamDuration, audioCodec, videoCodec, fileType, audioOnly, location) {
wscScripting.selectChoice(oQCheckUpload, oQCheckUpload.choices[0]);
}
//DESKTOP RECORDING STARTED EVENT
recorderInserted.onRecordingStarted = function (recorderId) {
wscScripting.selectChoice(oQCheckUpload, oQCheckUpload.choices[1]);
}
//DESKTOP video UPLOAD success event (different to RECORDING UPLOAD event)
recorderInserted.onDesktopVideoUploadSuccess = function (recorderId, filename, filetype, videoId, audioOnly, location) {
wscScripting.selectChoice(oQCheckUpload, oQCheckUpload.choices[0]);
}
//DESKTOP video UPLOAD started event
recorderInserted.onDesktopVideoUploadStarted = function (recorderId, filename, filetype, audioOnly) {
wscScripting.selectChoice(oQCheckUpload, oQCheckUpload.choices[1]);
}
//MOBILE video UPLOAD success event
recorderInserted.onVideoUploadSuccess = function (recorderId, filename, filetype, videoId, audioOnly, location) {
wscScripting.selectChoice(oQCheckUpload, oQCheckUpload.choices[0]);
}
//MOBILE video UPLOAD started event
recorderInserted.onVideoUploadStarted = function (recorderId, filename, filetype, audioOnly) {
wscScripting.selectChoice(oQCheckUpload, oQCheckUpload.choices[1]);
}
});
};
document.getElementsByTagName('head')[0].appendChild(oScript);
};
document.getElementsByTagName('head')[0].appendChild(oLink);
Match Choices and Have Pages Match That Randomisation
The script below will allow us to display pages generated with page looping in the same order as selected choices from a previous question, even when the choices have been randomised.
In the example survey link below, we have a multi checkbox question with randomised choices. The choices you have selected in the first question will then be displayed in the same order for the succeeding questions.
Click the following link to see an example:
Choice Randomisation & Page Looping Example.
//Get the question with the CHOICES we want to check
var oQuestion = wscScripting.getQuestionByDataPipingCode('FASTFOOD');
//Check if the question exists
if (oQuestion != null) {
//Get the question Choices
var aChoices = oQuestion.choices;
//Setup an array for the question choice tags to be pushed into
var aChoiceTagOrder = [];
//Loop through each choice in our question
for (var nChoice = 0; nChoice < aChoices.length; nChoice++) {
//Grab a new choice each loop
var oChoice = aChoices[nChoice];
//Grab the tag for that choice
var oTag = wscScripting.getTagValueByName(oChoice.tags, 'CODE');
//Push the tag text into the aChoiceTagOrder array
aChoiceTagOrder.push(oTag.text);
};
//We now need to find ONLY the randomised pages that match our BLOCK and add them to the array aRandomPages
//Get our survey pages
var aPages = wscScripting.getPageRandomizationItems();
//If the pages exist
if (aPages != null) {
//Create an array that will hold the pages we want
var aRandomPages = [];
//We need to know where to come back to
var nOrdinal = -1;
//Loop through the survey pages in the aPages array, if the page is randomized and belong to block1, push them into the aRandomPages array
for (var nPage = 0; nPage < aPages.length; nPage++) {
//Grab a survey page
var oPage = aPages[nPage];
//Check if page is randomised and is in BLOCK1
if (oPage.isRandom && oPage.blockCode == 'BLOCK1') {
//If a page is found that is randomised and from block1,
//push the page into the aRandomPages array
aRandomPages.push(oPage);
//Keep track of the ordinal integer
if (nOrdinal < 0) {
//When we update our order later, we don't want to start at first page which is 0 in the array
//nOridnal will be set to nPage after the first loop
nOrdinal = nPage;
};
};
};
//We now need to get our BLOCK pages in the aRandomPages array into the same order as the choices in our question
//Create an array that will hold the NEW order of the pages, this order will need to match the order of the choices in the question
var aCorrectPageOrder = [];
//Now to put them in the same order as the question choices
//Loop through the ORDER of the choices in the question - (we already setup the choice order as tags from earlier in the aChoiceTagOrder array)
for (var nChoice = 0; nChoice < aChoiceTagOrder.length; nChoice++) {
//Grab a choice tag from the ChoiceTagOrder array
var sChoiceTag = aChoiceTagOrder[nChoice];
//Loop through our pages in the aRandomPages array
for (var nPage = 0; nPage < aRandomPages.length; nPage++) {
//Grap a page
var oPage = aRandomPages[nPage];
///We need to find a page with a TITLE that matches the CHOICE TAG
//Eg: choice tag MACCAS and page title MACCAS
//Check if the randomised page TITLE matches the CHOICE TAG (both will be strings)
if (oPage.title == sChoiceTag) {
//If a choice tag and page title match, push that page into the aCorrectPageOrder array
aCorrectPageOrder.push(oPage);
};
};
};
//The pages in the aCorrectPageOrder array will now be the same as the order of the choices in the question - now we need to find the BLOCK and replace the pages
//Loop through the pages in the correctly ordered array
for (var nPage = 0; nPage < aCorrectPageOrder.length; nPage++) {
//Grab a page object from the new page order
var oPage = aCorrectPageOrder[nPage];
//We can now start updating our page order in the aPages array, start updating at ordinal 1
//In the array, we don't want to update the first page
aPages[nOrdinal] = oPage;
//Add +1 to the ordinal each time a page is added
nOrdinal = nOrdinal + 1;
};
//Once all the correct pages have been updated in the aPages array, we can now set the new page order
wscScripting.setPageRandomizationItems(aPages);
};
};
This script should be placed in the Execute Javascript to Test Randomization Parameters prior to Next Button scripting event.
Hide a Cell in a Single Choice Matrix Based Off the Choice Value & Row Tag
The script below will disable a cell in a Single Choice Matrix Question. A tag will need to be applied to each row in the matrix to gain access to the row with scripting. EG: ROW:1, ROW:2 etc.
You will need to gain access to your question, the row and the value of the choice to disable your desired cell in the matrix.
///Get the Question
var oMatrixQuestion = wscScripting.getQuestionByDataPipingCode('MATRIX');
///Get the choice you want to disable by using it's value
var oChoice = wscScripting.getChoiceByValue(oMatrixQuestion, 1);
///Get the first row in the matrix
var oRow = wscScripting.getRowByTagValue(oMatrixQuestion, 'ROW', '1');
///Use the question, the choice and the row to disable the cell using the hideMatrixCell method
wscScripting.hideMatrixCell(oMatrixQuestion, oChoice, oRow);
This script should be placed in the Execute Javascript when Survey Page has Loaded scripting event.
Allow Text Questions as Comments for Only the Top 3 Choices in a Ranking Question
This script will allow text questions to appear as comments for the top 3 selected choices in a ranking type question. The text questions will only be displayed if their corresponding choice is selected in the top 3 for the ranking question, otherwise they will remain hidden.
You will need to ensure that you have the same amount of text questions and ranking choices. So if you had 6 ranking choices, you will need 6 text questions for each choice. Currently the script targets each text question with an access code of 'TEXT' followed by a choice value from the ranking choices. EG: TEXT1, TEXT2. The access codes can be changed by adjusting the variables var oText.
The following code will need to be adjusted based on how many choices you will have in your ranking question:
for (var nText = 1; nText <= 6; nText++), the integer of 6 is set for a ranking question of 6 text questions that need to be looped through. If you have more or less you can adjust this integer.
To view an example:
Click Here
$(document).ready(function(){
//Get the ranking question
var oQuestion = wscScripting.getQuestionByDataPipingCode('RANKING');
//Find the target question element
var target = document.querySelector('#' + oQuestion.identity + '_rankedcontainer');
//Create an observer instance
var observer = new MutationObserver(function (mutations) {
mutations.forEach(function (mutation) {
//Get the ranking question
var oQuestion = wscScripting.getQuestionByDataPipingCode('RANKING');
//Get all the ranks
var aRanks = wscScripting.getSelectedRanks(oQuestion);
//Keep a list of each of the top three ranks
var aValues = new Array();
//Check each of the ranks
for (var nRank = 0; nRank < 3; nRank++) {
//If nRand is less than the aRanks array
if (nRank < aRanks.length) {
//Get a selected choice from the ranks aRanks array
var oRank = aRanks[nRank];
//Show the question if it's ok
aValues.push(oRank.value.toString());
};
};
//Loop through the question choices
for (var nChoice = 0; nChoice < oQuestion.choices.length; nChoice++) {
//Get the value of the choice, convert it to a
string
var sValue =
oQuestion.choices[nChoice].value.toString();
//Get the text question, add the value of the choice
to target the matching question
var oText =
wscScripting.getQuestionByDataPipingCode('TEXT' + sValue);
//Check if the question exists
if (oText != null) {
//Check sValue exists in the aValues
array
if ($.inArray(sValue, aValues) > -1) {
//If oRank.value is found in the aValues array, show
the question
wscScripting.showQuestion(oText);
} else {
wscScripting.hideQuestion(oText);
wscScripting.setValue(oText, '');
};
};
};
});
});
//Configuration for the observer:
var config = {
attributes: true,
childList: true,
characterData: true
};
//Pass in the target node and the observer options
observer.observe(target, config);
});
//Loop through the text questions and hide them
for (var nText = 1;
nText <= 6; nText++) {
var oText = wscScripting.getQuestionByDataPipingCode('TEXT' + nText.toString());
if (oText != null) {
//Hide the question
wscScripting.hideQuestion(oText);
};
};
This script should be placed in the Execute Javascript when Survey Page has Loaded scripting event.
Drag Drop Flags Horizontal Bar with Three Style Gradient Colours
The following script can be used to customise the colours of the Drag Drop Flags question type horizontal options bar.
The script simply uses Jquery and the question identity to modify the linear gradient of the bar. Colours can be passed in as a string or a variable holding a string colour value.
//Get the question
var oQuestion = wscScripting.getQuestionByDataPipingCode('FLAGS');
//Get the question div element identity
var sFlagsIdentity = oQuestion.identity;
sFlagsIdentity = "ruler_" + sFlagsIdentity;
//Create a three style gradient
$('#' + sFlagsIdentity).css("background-image", "linear-gradient(to right, #FF0000, #FF0000, #FF0000, #D6E1EB, #D6E1EB, #FF00FF, #FF00FF, #FF00FF)");
Get a Drag Drop Flag Location Value
This script will allow you to access the number value for each location that a flag is placed on the horizontal bar in a Drag Drop Flag question type. Each flag is represented as a row.
The example script below uses the question identity, question row identity and the question choice identity to access the value and parse it. A different row can be accessed by changing the oQuestion.rows[0].identity integer.
//Get the question
var oQuestion = wscScripting.getQuestionByDataPipingCode('FLAGS');
//Get the number value for each flag location
var nA = parseFloat($('#' + oQuestion.identity + '_' + oQuestion.rows[0].identity + '_' + oQuestion.choices[0].identity).val());
var nB = parseFloat($('#' + oQuestion.identity + '_' + oQuestion.rows[1].identity + '_' + oQuestion.choices[0].identity).val());
var nC = parseFloat($('#' + oQuestion.identity + '_' + oQuestion.rows[2].identity + '_' + oQuestion.choices[0].identity).val());
var nD = parseFloat($('#' + oQuestion.identity + '_' + oQuestion.rows[3].identity + '_' + oQuestion.choices[0].identity).val());
Ranking Question With Page Reorder
While quite tricky to do, it is possible to reorder survey pages based on selected ranked choices in a ranking type question.
For example, depending on the order of ranked choices that a respondent has selected, subsequent matching survey pages will be displayed in the same order.
To achieve this, a combination of scripting, survey flow and page randomisation is used. Pages that are to be matched with chosen ranked choices are set to be randomised, each with their own block code. Randomising the pages sounds strange and quite the opposite of the goal, however pages are only randomised for the purpose of identifying them in script.
Survey flow is used to hide pages that are not selected by the respondent. An extra hidden, multiple choice checkboxes question will be used in the survey with identical choices to the ranking question. This extra hidden question will be selected with script and used for triggering the page flow.
Click here to view an example
Two scripts are required for the example to work:
Script one will: get the selected ranking choices, then select matching choices in the extra multi checkbox question. This question will be used for page flow and to determine which choices were not selected. This script will be set to the Next or Submit Buttons are Pressed scripting event.
Script two will: Get the survey pages and reorder according to the ranking choice order. This script will be set to the Randomization Parameters are Tested prior to Next Button scripting event.
//Script One
//Select chosen rank choices in extra hidden question for page flow
//Get the questions
var oQRanking = wscScripting.getQuestionByDataPipingCode('RANKINGQ');
var oQHidden = wscScripting.getQuestionByDataPipingCode('SELECTEDCHOICES');
//Get the choices selected in the ranking question
var oSelectedChoices = wscScripting.getSelectedRanks(oQRanking);
//Loop through the select ranked selected choices
for (i = 0; i < oSelectedChoices.length; i++) {
//Select the same choices in the hidden question
wscScripting.selectChoiceByValue(oQHidden, oSelectedChoices[i].value);
};
//Script Two
//Get the questions
var oQRanking = wscScripting.getQuestionByDataPipingCode('RANKINGQ');
var oQHidden = wscScripting.getQuestionByDataPipingCode('SELECTEDCHOICES');
//Get the choices selected in the ranking question
var oSelectedChoices = wscScripting.getSelectedRanks(oQRanking);
//Get the survey pages
var aPages = wscScripting.getPageRandomizationItems();
//Get the pages that are random and push into the random pages array
var aRandomPages = [];
for (i = 0; i < aPages.length; i++) {
//Check if the page is random
if (aPages[i].isRandom == true) {
aRandomPages.push(aPages[i]);
};
};
//Get only the random pages that have matching codes and titles then push them into the aNewOrder array
var aNewOrder = [];
//Loop through the selected rank choices
for (i = 0; i < oSelectedChoices.length; i++) {
//Get a choice code
var sChoiceCode = oSelectedChoices[i].code;
//Loop through the random pages array
for (e = 0; e < aRandomPages.length; e++) {
//After getting a page, get the page title
var sRandomPageTitle = aRandomPages[e].title;
//Check if a rank choice CODE and a page TITLE match
if (sChoiceCode == sRandomPageTitle) {
aNewOrder.push(aRandomPages[e]);
};
};
};
//Get the choices that are NOT selected in the hidden question
var aNonSelectedChoices = [];
for (i = 0; i < oQHidden.choices.length; i++) {
if (wscScripting.isChoiceSelected(oQHidden, oQHidden.choices[i]) == false) {
//Push a non selected choice into the aNonSelectedChoices array
aNonSelectedChoices.push(oQHidden.choices[i]);
};
};
//Get the pages of the non selected choices, push them to the end of the new order
for (i = 0; i < aNonSelectedChoices.length; i++) {
//Get a choice code
var sChoiceCode = aNonSelectedChoices[i].code;
//Loop through random pages
for (e = 0; e < aRandomPages.length; e++) {
var sRandomPageTitle = aRandomPages[e].title;
//Check if the choice CODE and TITLE match
if (sChoiceCode == sRandomPageTitle) {
aNewOrder.push(aRandomPages[e]);
};
};
};
//Setup the final order and set page randomization items
//Get the non random pages
var aNonRandomPages = [];
for (i = 0; i < aPages.length; i++) {
//Check if the page is random, only push the pages that are not random
if (aPages[i].isRandom == false) {
aNonRandomPages.push(aPages[i]);
};
};
//Add the non random pages back to the array
aNewOrder.unshift(aNonRandomPages[0]);
aNewOrder.push(aNonRandomPages[1]);
//Set the new order
wscScripting.setPageRandomizationItems(aNewOrder);
Retrieve Meta Data from a Text List Question
This script will parse a JSON package from a Text List question type. After parsing the package, you can access the data by using javascript dot or bracket notation.
//Get the question
var oQuestion = wscScripting.getQuestionByDataPipingCode('TEXTLIST');
//Get the question value
var oMeta = $('#' + oQuestion.identity + '_metadata').val();
//Parse the JSON package
oMeta = JSON.parse(oMeta);
//Access the data
oMeta["State"];
oMeta.State;
Hide a Question Based on if a Terms and Conditions Checkbox is Checked
Sometimes you may want to hide specific questions on a survey page if the respondent clicks on a Terms and Conditions Checkbox.
This can be done using an on click scripting event, which will check the state of the checkbox when clicked and then execute code to hide or show question.
To identify if a Terms and Conditions checkbox is checked, the wscScripting.getValue() method must be used. This method will return false if the checkbox is not checked, or true if the checkbox is checked.
//Get the question that will be hidden
var oQuestion = wscScripting.getQuestionByDataPipingCode('QUESTION');
//Terms and Conditions (Single Checkbox)
var oTermsQuestion = wscScripting.getQuestionByDataPipingCode('TERMS');
//Setup the on click function on the terms and conditions question
$('#' + oTermsQuestion.identity).on('click', function () {
//Check if the terms/conditions box is checked
var termsBox = wscScripting.getValue(oTermsQuestion);
//Check if the terms and conditions box was NOT checked
if (termsBox == false) {
//If the box is not checked, show the question
wscScripting.showQuestion(oQuestion);
wscScripting.enableQuestion(oQuestion);
//Check if the terms and conditions box was WAS checked
} else if (termsBox == true) {
//If the box is checked, hide both the numeric and text question, reset the questions
wscScripting.hideQuestion(oQuestion);
wscScripting.disableQuestion(oQuestion);
//Reset the question, this remove text and choice data for the hidden question
wscScripting.resetQuestion(oQuestion);
};
});
Retrieve Data from the Spoonacular API using AJAX
The Spoonacular API offers a variety of food based data that can be retrieved via Javascript API calls.
The Spoonacular API documentation offers an array of different GET or POST request methods for retrieving data - before you can start making requests you will need to sign up for an API key. The key will be used to track requests made with the API.
Use the following link to view the Spoonacular API documentation:
Spoonacular Documentation
store the
The below script is a basic example of retrieving data from the API using a HTTP GET request with AJAX. Returned data will be in a JSON format, which can be saved into a variable. The maximum number of returned results can be changed by adjusting the "number" parameter.
//Setup a variable to hold the data returned data
var aIngredients;
//Setup the AJAX function for making an API GET request
$.ajax({
type: "GET",
async: false,
url: "https://api.spoonacular.com/food/ingredients/search?apiKey=insert-your-api-key-here",
data: { "query": "banana", "metaInformation": true, "number": 3 }
}).done(function(data) {
//Assign the returned data to the variable
//Other logic and functions can be written here after the request is done
var x = data;
aIngredients = x;
});
//View the data in the console
console.log(aIngredients.results[0]);
console.log(aIngredients.results[1]);
console.log(aIngredients.results[2]);
Drag Drop Flags - Horizontal Bar with Custom Icons and Height
The Drag Drop Flags question type allows for respondents to place flags onto a horizontal score bar, this bar can be
manipulated with JavaScript to adjust the height and allow for icons at each end of the bar.
EG:

Use the script below to add image icons to the left and right hand side of the horizontal bar. The width and height
of the image html tag attributes may need to be adjusted to appropriately fit the bar. EG: style="width: 30px;
height: 30px;".
Ensure that your question has a valid Question Access Code and the script is set to the "execute when the Survey
Page Has Loaded" scripting event.
//Get the question
var oQuestion = wscScripting.getQuestionByDataPipingCode('FLAGS');
//Adjust the bar height
$('#ruler_' + oQuestion.identity).height(40);
$('#ruler_' + oQuestion.identity + ' .tick').height(30)
//Add image tags to the horizontal bar
$('#ruler_' + oQuestion.identity).append($('<div style="left:2px;top:5px;position:absolute;"><img src="PUT-YOUR-IMAGE-URL-HERE"></div>'));
$('#ruler_' + oQuestion.identity).append($('<div style="right:2px;top:5px;position:absolute;"><img src="PUT-YOUR-IMAGE-URL-HERE"></div>'));
Render a Multiple Choice, Checkboxes Question as a 3x3 Grid on Mobile
The following script will force a Multiple Choice Checkbox question to render as a 3x3 grid on mobile devices, rather than having choices stacked in a single column. Nine selection choices will be needed in the question for this script to function.
EG:

Try an example on your mobile:
Here
//Check if the user is on a mobile device
if (wscScripting.getDisplayType() == 'mobile') {
//Get the question
var oQuestion = wscScripting.getQuestionByDataPipingCode('IMAGES');
//Create the new table elements
$('#' + oQuestion.identity + '_div')
.append($('<table>')
.attr('id', oQuestion.identity + '_table2')
.append($('<tr>')
.append($('<td>')
.attr('class', 'cell_11'))
.append($('<td>')
.attr('class', 'cell_12'))
.append($('<td>')
.attr('class', 'cell_13')))
.append($('<tr>')
.append($('<td>')
.attr('class', 'cell_21'))
.append($('<td>')
.attr('class', 'cell_22'))
.append($('<td>')
.attr('class', 'cell_23')))
.append($('<tr>')
.append($('<td>')
.attr('class', 'cell_31'))
.append($('<td>')
.attr('class', 'cell_32'))
.append($('<td>')
.attr('class', 'cell_33'))));
//Grab array of table items
var aItems = $('#' + oQuestion.identity + ' tr table');
var nRow = 1;
var nChoice = 1;
//Loop through the items
for (var nItem = 0; nItem < aItems.length; nItem++) {
$('#' + oQuestion.identity + '_table2 .cell_' + (nRow.toString()) + (nChoice.toString())).append($(aItems[nItem]).detach());
nChoice++;
if (nChoice > 3) {
nChoice = 1;
nRow++;
}
}
//Hide the old elements
$('#' + oQuestion.identity).hide();
//Adjust the element height
$('#' + oQuestion.identity + '_table2 tr table').css('height', '');
}
Store the Browser Local Date and Time in a Hidden Text Question
Javascript can be used to grab the local date, time and timezone from a respondent web browser during a survey. This value can then be stored in a hidden question on the survey page.
The following script can be used to store the data in a text input question:
//Get the text question
var oQTime = wscScripting.getQuestionByDataPipingCode('TIME');
//Create a new date object
var oDate = new Date();
var nMonth = oDate.getMonth() + 1;
var sMonth = nMonth.toString();
if (sMonth.length == 1) {
sMonth = '0' + sMonth;
}
var nDay = oDate.getDate();
var sDay = nDay.toString();
if (sDay.length == 1) {
sDay = '0' + sDay;
}
var nYear = oDate.getFullYear();
var nHours = oDate.getHours();
var sHours = nHours.toString();
if (sHours.length == 1) {
sHours = '0' + sHours;
}
var nMinutes = oDate.getMinutes();
var sMinutes = nMinutes.toString();
if (sMinutes.length == 1) {
sMinutes = '0' + sMinutes;
}
var nSeconds = oDate.getSeconds();
var sSeconds = nSeconds.toString();
if (sSeconds.length == 1) {
sSeconds = '0' + sSeconds;
}
//Combine the values and create a readable format
sDate = nYear.toString() + '-' + sMonth + '-' + sDay + ' ' + sHours + ':' + sMinutes + ':' + sSeconds;
var nTimeZone = -oDate.getTimezoneOffset()/60;
var sTimeZone = 'GMT'
if (nTimeZone > 0) {
sTimeZone = 'GMT+' + nTimeZone;
}
else if (nTimeZone < 0) {
sTimeZone = 'GMT-' + nTimeZone;
}
sDate = sDate + ' ' + sTimeZone;
//Set the local time and data value into the question
wscScripting.setValue(oQTime, sDate);
Store the Length of Time Taken to Select Choices for a Downscaled Matrix
Javascript can be used to record record time lengths during a survey. This example demonstrates recording how long
it takes for a user to select a choice in a downscaled Matrix question. The value is then stored into a numeric grid
which can be hidden on the page.
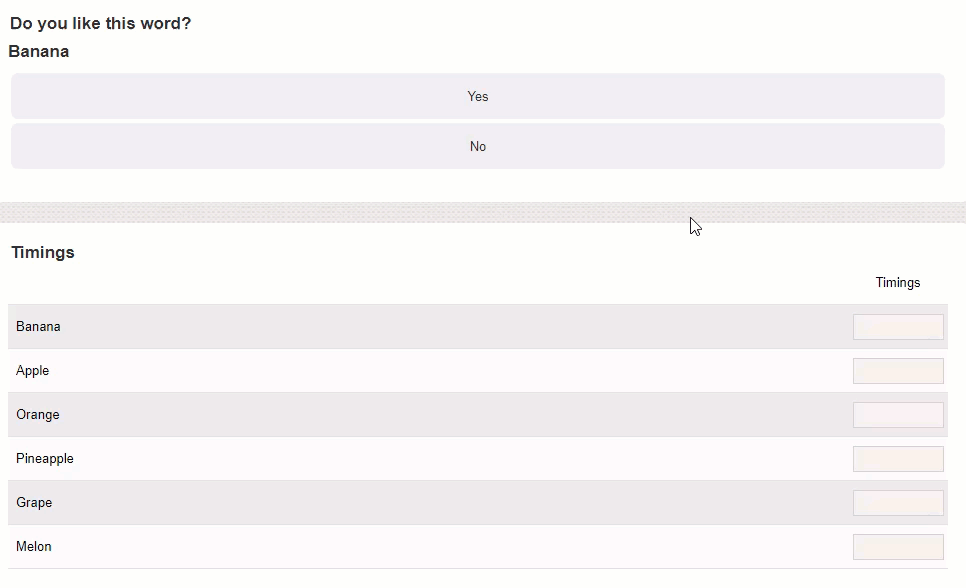
Here are the steps for setting up the questions:
1. Add a Radio Buttons Matrix question to the survey page. Ensure that all the question rows have a CODE TAG. EG,
CODE:BANANA.
2. Set the Mobile Device Display Options to "Force render of Question on all devices using Mobile Options". Use the
"Show Only Current Row with Click & Show Next Row [Radio Buttons, Forward Direction Only]" Mobile Display
Method.
3. Add a Numeric Grid question to the survey page. Copy the rows from your matrix question into your numeric grid,
ensure they have matching row TAGS for both questions. Add one choice to the numeric grid. Set the Numeric Format to
"Numbers with Decimals".
You can then add the following script to the page, set the script to the "Execute Javascript when Survey Page has
Loaded" scripting event.
function SaveTime(sId) {
///Get the matrix question
let oQuestion = wscScripting.getQuestionByDataPipingCode('MATRIX');
///Get a row from the matrix question
let oRow = wscScripting.getRowByTagValue(oQuestion, 'CODE', sId);
///Create a new date object
let oCurrent = new Date();
///Calculate the new date time minus the window matrix current time
let timeDiff = oCurrent - window.MatrixCurrentTime;
///Remove the milliseconds from the time
timeDiff /= 1000;
///Get the numeric grid question
let oTime = wscScripting.getQuestionByDataPipingCode('TIMING');
///Get the tag value from oRow by name
let oTag = wscScripting.getTagValueByName(oRow.tags,'CODE');
///Get the row form the numeric grid question
let oTimeRow = wscScripting.getRowByTagValue(oTime, 'CODE', oTag.text);
///Get the current value of the numeric grid question row
let nCurrentValue = wscScripting.getMatrixValue(oTime, oTime.choices[0], oTimeRow);
///Set the timed value into the numeric grid
///Only update the numeric grid time if has not been updated before, once set dont set it again
if (isNaN(nCurrentValue)) {
wscScripting.setMatrixValue(oTime, oTime.choices[0], oTimeRow, timeDiff);
window.MatrixCurrentTime = new Date();
}
///Keep track of the selected matrix choices
let nCount = 0;
///Set the render size element to mobile
$('#RenderSize').val('mobile');
///Get the number of selected choices for each row, add to nCount
for (let nRow = 0; nRow < oQuestion.rows.length; nRow++) {
let oRow = oQuestion.rows[nRow];
let aChoices = wscScripting.getSelectedMatrixChoices(oQuestion, oRow);
///If a choice is selected, add it to nCount
if (aChoices.length > 0) {
nCount++;
}
}
///If the number of choices selected matches the number of rows, there are no more items to rate
if (nCount == oQuestion.rows.length) {
for (let nRow = 0; nRow < oQuestion.rows.length; nRow++) {
let oRow = oQuestion.rows[nRow];
if (nRow == 0) {
if ($('#' + oQuestion.identity + '_norater').length == 0) {
$('#' + oQuestion.identity + '_' + oRow.identity + '_0_title').parent().append('<div id="' + oQuestion.identity + '_norater">There are no further items to rate.</div>');
$(('#' + oQuestion.identity + '_norater').css('width','100%');
$('#' + oQuestion.identity + '_norater').css('text-align','center');
$('#' + oQuestion.identity + '_norater').css('height','75px');
$('#' + oQuestion.identity + '_norater').css('padding-top','50px');
}
}
$('#' + oQuestion.identity + '_' + oRow.identity + '_0_title').hide();
$('#' + oQuestion.identity + '_' + oRow.identity + '_0_body').hide();
}
}
}
///Get the matrix question
let oQuestion = wscScripting.getQuestionByDataPipingCode('MATRIX');
///Loop through the matrix question rows and choices
for (let nRow = 0; nRow < oQuestion.rows.length; nRow++) {
let oRow = oQuestion.rows[nRow];
for (let nChoice = 0; nChoice < oQuestion.choices.length; nChoice++) {
let oChoice = oQuestion.choices[nChoice];
let oTag = wscScripting.getTagValueByName(oRow.tags,'CODE');
let sTag = oTag.text;
///Create the click event that will call the SaveTime function
$('#' + oQuestion.identity + '_' + oRow.identity + '_0_' + oChoice.identity + '_table').on('click', function() {
let sValue = sTag;
///Pass the string tag value of the choice that was clicked on to the function
SaveTime(sValue);
});
}
}
Get the Age of a Respondent from a Date Question
The getAgeFromDate() scripting method can be used to obtain how old a respondent is based on their date of birth
entered into a Date Question. The age of the respondent can then be stored in a Numeric question.
The following code will capture the age value when set to the On Next or Submit scripting event:
//Get the date question
var question = wscScripting.getQuestionByDataPipingCode('DOB');
if (question) {
//Get the value entered into the date question
var getValue = wscScripting.getValue(question);
//Pass the date value to the getAgeFromDate method
var age = wscScripting.getAgeFromDate(getValue);
//Get the numeric question
var myage = wscScripting.getQuestionByDataPipingCode('AGE');
//Set the age value into the numeric question
wscScripting.setValue(myage, age);
}
Select a Question Choice if no Choices have been Selected On Next or Submit
The isAnyChoiceSelected() scripting method can be used to determine if no choices have been selected for a choice
question. You can pass in a question and it's choices as arguments, the method will return false if no choices have
selected for the choice question.
The returned boolean value can then be used in scripting logic to select a question choice(s) only if the question
had no choices selected, this code can then be executed during the On Next or Submit scripting event:
///Get the question choice question
var oQ = wscScripting.getQuestionByDataPipingCode('MYCHOICEQUESTION');
///Determine if any choices have been selected, store the true or false value in a variable
var bAreChoicesSelected = wscScripting.isAnyChoiceSelected(oQ, oQ.choices);
///Run a test, if the returned value was false, no choices have been selected for the question
if (bAreChoicesSelected == false) {
///Execute some code, select a choice by it's value
wscScripting.selectChoiceByValue(oQ, 1);
};
Hide/Show an Other Specify Text Question when Other is Selected in a Hierarchical List Question
Use the script below to hide/show an other specify text question when other is selected in a hierachical list
question.
///Get the Other Specify text question
var oOther = wscScripting.getQuestionByDataPipingCode('OTHER');
///Hide the Other Specify text question when the respondent enters the page
wscScripting.hideQuestion(oOther);
///Get the Hierarchical List question
var oQuestion = wscScripting.getQuestionByDataPipingCode('MYLIST');
///If the question exists
if (oQuestion) {
///Setup the on change function, execute the code only when the list is changed
$('#' + oQuestion.identity + '_1').on('change', function () {
///Get the Hierarchical List question
var oQ = wscScripting.getQuestionByDataPipingCode('MYLIST');
///Get the Other Specify text question
var oOther = wscScripting.getQuestionByDataPipingCode('OTHER');
///Get the string/string value of the selected list choice
var sValue = $('#' + oQ.identity + '_1 option:selected').text();
///If Other is selected
if (sValue == 'Other') {
///Show the Other Specify text question
wscScripting.showQuestion(oOther);
} else {
///Hide the Other Specify question
wscScripting.hideQuestion(oOther);
}
});
};
Auto Select the Current Day in a Choice Question
Use the script below to auto select the current day of the week in a single choice question.
A single choice question can be setup with the following days of the week and assigned values:
- Sunday|1
- Monday|2
- Tuesday|3
- Wednesday|4
- Thursday|5
- Friday|6
- Saturday|7
The JavaScript getDay() method can be used to aquire the current day from a Date object. It is important to note
that the getDay() method returns the day of the week as values (0 to 6).
///Get the choice question
var oQuestion = wscScripting.getQuestionByDataPipingCode('CHOICEQUESTION');
///Create a new date object
var oDate = new Date();
///Get the current day value from the date object using the getDay() JavaScript method
var nDay = oDate.getDay();
/// Days in 'CHOICEQUESTION' are Sunday to Saturday with the values 1 - 7
///Javascript days start at Sunday = 0, Monday = 1 etc
///Therefore when selecting the the choice by value, add +1 to the nDay variable
wscScripting.selectChoiceByValue(oQuestion, nDay + 1);
Prevent a respondent from Clicking Next for some time
Use both the scripts below to prevent a respondent from click next until a certain amount of time has passed.
The first script is a Page Load that sets up the monitor.
/// Loaded Time
window.pageLoadTime = Date.now();
/// Load a 30 second timer
setTimeout(function() {
CheckNextVisibleStatus();
}, 30000);
The second script is a Next or Submit Button Visibility is Tested script that checks the monitor
/// Loaded Time
var nOldTime = window.pageLoadTime;
/// Curent Time
var nTime = Date.now();
/// More than 30 seconds
args.isValid = ((nTime - nOldTime) > 30000);